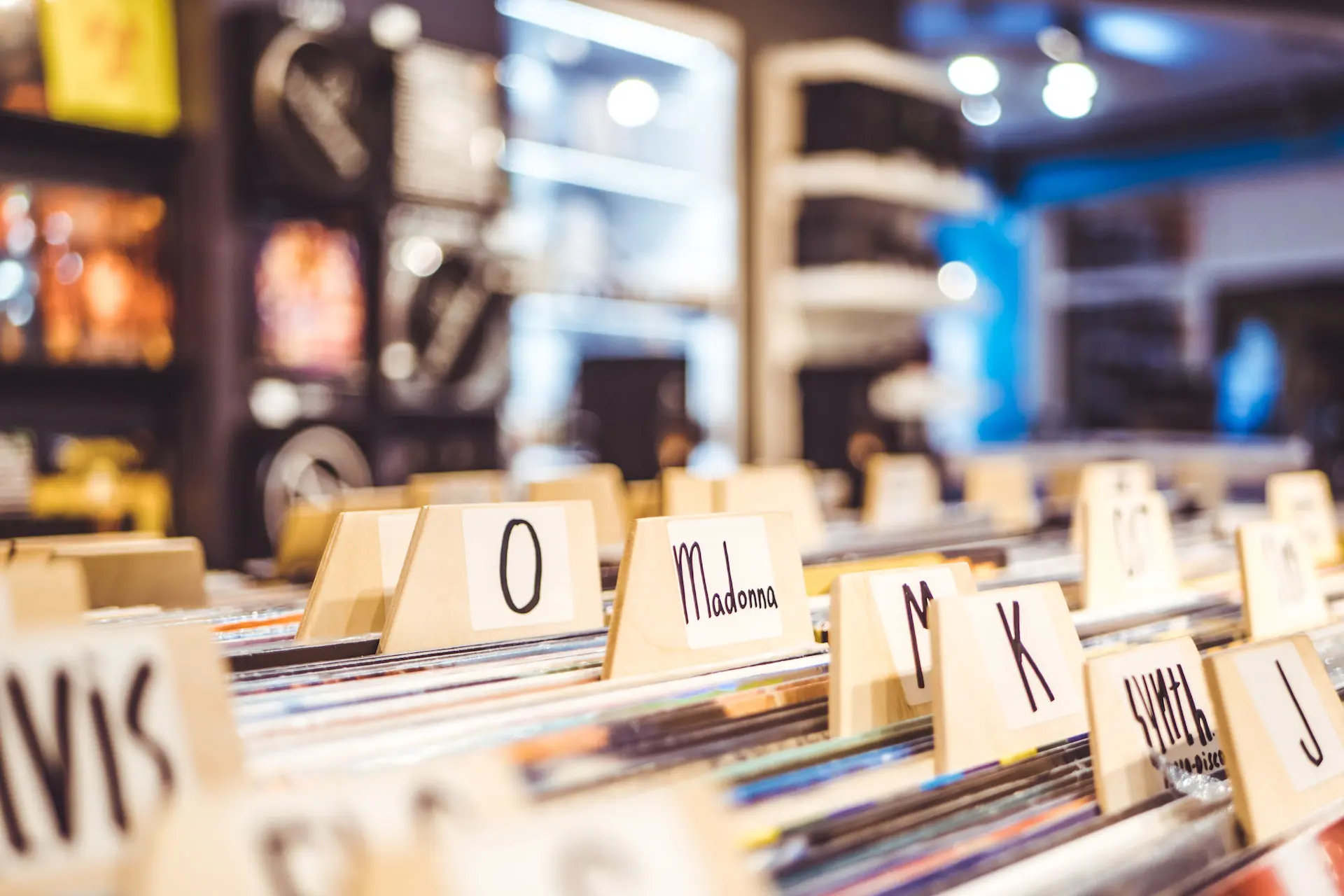
Recursively List Files in a Directory With Python
Get a list of all files in a given directory
Use the os
library to recursively walk
through a given directory.
import os
def getAllFilesInDirectory(directory):
all_files = []
# os.walk() returns an iterator, a tuple with 3 elements: the root dir, [dirs], [files]
for i in os.walk(directory):
files_in_current_directory = i[2]
for file in files_in_current_directory:
all_files.append(file)
return all_files
Get a list of all files with their paths jusin a given directory
The tuple returned by os.walk()
also contains the directory the files are in. We can use this information to get the full file paths of every file in a given directory.
import os
def getAllFilesInDirectoryWithPath(directory):
all_files_with_path = []
# os.walk() returns an iterator, a tuple with 3 elements: the root dir, [dirs], [files]
for i in os.walk(directory):
current_directory = i[0]
files_in_current_directory = i[2]
for file in files_in_current_directory:
file_path = f'{current_directory}/{file}'
all_files_with_path.append(file_path)
return all_files_with_path